Most Commonly Asked Python Usecases - Part 4 (OOPs Concepts)
- Neema MV
- Jul 26, 2021
- 3 min read
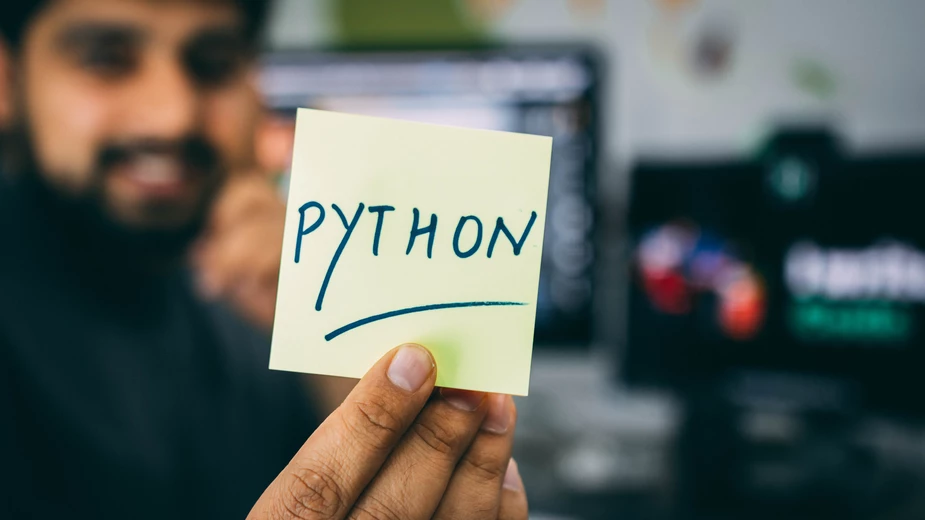
Photo by Hitesh Choudhary on Unsplash
Hi All! Hope you all had a great weekend!! This is the part 4 of the most commonly asked python usecases. Last week, we saw in Part 3 python usecases on data visualization. Previously, we looked into Part 2 python usecases on Pandas & Part 1 python concepts such as lists, list comprehensions, numpy array, sets, dictionaries, etc. Python is great programming language that allows Object Oriented Programming. It helps us to create a class with attributes and methods. If you understand OOP you have a much deeper understanding of Python and programming in general. And so this week, we are going to look at Python OOPs concepts in the form of case study. Dont miss the bonus content below.
Most Commonly Asked Python OOPs Concepts:
1. Develop a class with a message: In the below example, a bank class created and displays the address of the account holder.
class Bank:
def __init__(self, firstname, lastname, address,accounttype):
self.firstname = firstname
self.lastname = lastname
self.address = address
self.accounttype = accounttype
def show_address(self):
return "Address given is {}". format(self.address)
bank1 = Bank("Neema", "MV", "Chennai", "savings")
print(bank1.show_address())
Address given is Chennai
2. Show an example of an inheritence method using the above Bank Class:
class Citibank(Bank):
def __init__(self, firstname, lastname, address,accounttype,bank_home_branch):
super().__init__(firstname, lastname, address,accounttype)
self.bank_home_branch = bank_home_branch
def show_address(self):
print("Citibank account holder address is shown here")
citi_bnk = Citibank("Sara", "Cally", "Delhi", "savings", "Chennai")
citi_bnk.bank_home_branch
'Chennai'
As you can see, the Citibank class uses the parent class 'Bank' and has additional method 'bank_home_branch'. Rest of the methods are from its parent class.
3. Show an example of the Polymoriphism using the above class:
citi_bnk.show_address()
Citibank account holder address is shown here
When you call the method the show_address method is called for the child class instead of the Parent class and this is called 'Polymorphisim'.
4. Update the inbuilt __str__ method witha new custom message:
Note: Magic methods are those inbuilt methods when a class is created. In the below example, we can how to override a magic method "__str__"
class Bank:
def __init__(self, firstname, lastname, address,accounttype):
self.firstname = firstname
self.lastname = lastname
self.address = address
self.accounttype = accounttype
def __str__(self):
return "The object is initialized"
def __sizeof__(self):
return "The size of the object is shown here"
def show_address(self):
return "Address given is {}". format(self.address)
#updateing method __str__()
b = Bank("Neema", "MV", "Chennai", "savings")
print(b)
The object is initialized
Here, you can see that the __str__ method is overriden with the message 'The object is initialized'.
5. How do you update the change the email address when the lastname of the account holder after marriage.
class Bank:
def __init__(self, firstname, lastname, address,accounttype):
self.firstname = firstname
self.lastname = lastname
self.address = address
self.accounttype = accounttype
@property
def email(self):
return self.firstname+"_"+self.lastname+"@gmail.com"
def __str__(self):
return "The object is initialized"
def __sizeof__(self):
return "The size of the object is shown here"
def show_address(self):
return "Address given is {}". format(self.address)
bank_obj = Bank("Sheila", "N", "Delhi", "savings")
bank_obj.email
'Sheila_N@gmail.com'
bank_obj.lastname = "KH"
bank_obj.email
'Sheila_KH@gmail.com'
As you can see, with the use of property decorator we can change the behavior of email , email(self) is a method but it operates a normal property. this special method is called Getter and Setter.
Bonus:
1. Empty class is a bad way to define a class.
class car:
pass
2. __init__ Acts like a constructor
3. Class has a predefined name, __init__, and does not have a return value
4. if you type in the code dir(objectofclass), you can find many magic method including the methods that you have created for your class.
5. Decorators are very powerful and useful tool in Python since it allows programmers to modify the behavior of function or class. (Source: GeeksforGeeks)
Best Sources:
This marks the end of the Python Series. If you like this content, please like, share and subscribe!!
Top Viewed articles:
Kommentare