Most Commonly Asked Python Usecases - Part 1 (Python Basics)
- Neema MV
- Jul 5, 2021
- 4 min read
Updated: Jul 19, 2021
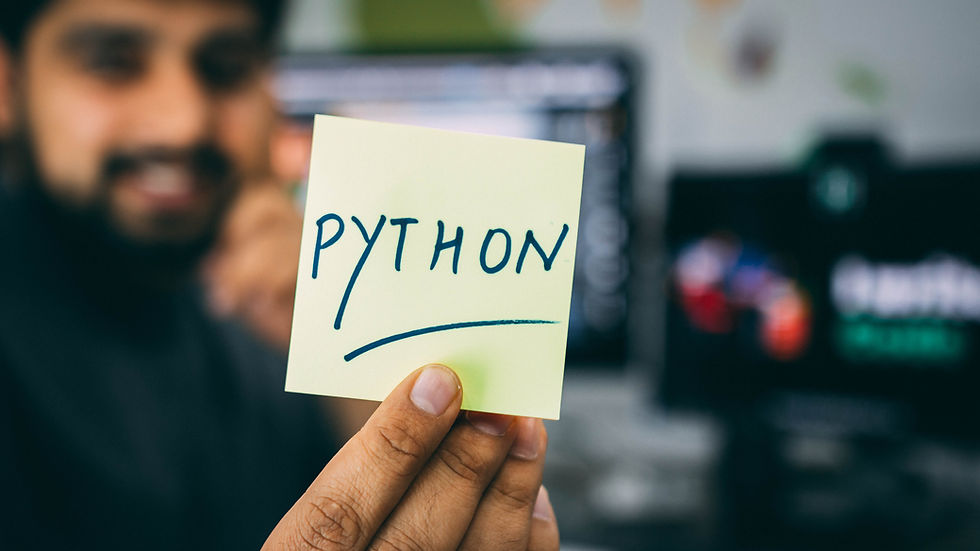
Photo by Hitesh Choudhary on Unsplash
Hi All!! Hope you have a great weekend!! Python as a programming language is widely talked about and practiced in the field of Data Science. As I was going through various interview topics, I found many tutorials that explain Python topics/concepts. But I was preferring to see some use cases where we can apply the concepts. I have collated the widely commonly asked Python interview use cases:
Most commonly asked Python topics and use cases
Lists:
1. Program to square of the list values:
#Lists
#1. Program to square of the list values
lst = [1,2,3,4]
#using simple function
def sum_list(lst):
ret=[]
for i in lst:
ret.appen(1**2)
return ret
output = sum_array(lst)
print(output)
[1,4,9,16]
2. Square of the list values using map & lambda function:
output = list(map(lambda a:a**2, lst))
print(output)
[1,4,9,16]
3. Filter only the vowels:
input = ['a','b','c','d','e','f']
vowel_list = ['a','e','i','o','u']
output = list(filter(lambda a: a in vowel_list, input))
print(output)
['a','e']
4. rotation of list:
lst = [1,2,3,4,5,6]
def rot_array(lst, num):
#using list slicing
temp = lst[0:num]
fin_lst = lst[num:]+temp
return fin_lst
output = rot_array(lst,2)
print(output)
[3, 4, 5, 6, 1, 2]
5. smallest and largest of the list:
a = [5, 10, 15, 20, 25]
[a[0], a[-1]]
[5, 25]
Related concepts: List comprehension,for loop, map, lambda, filter and list indexing
Numpy Array
1. Replace colunm 2 and replace with new column:
import numpy as np
input_arr = np.array([[10,20,30],[40,50,60],[70,80,90]])
print("Input Array:")
print("Array after deleting column 2 on axis 1")
input_arr = np.delete(input_arr , 1, axis = 1)
print (input_arr)
new_arr = np.array([[10,10,10]])
print("Array after inserting column 2 on axis 1")
input_arr = np.insert(input_arr , 1, new_arr, axis = 1)
print (input_arr)
Input Array:
Array after deleting column 2 on axis 1
[[10 30]
[40 60]
[70 90]]
Array after inserting column 2 on axis 1
[[10 10 30]
[40 10 60]
[70 10 90]]
2. Print zeros with 2 rows and 3 columns:
np.zeros((2,3))
array([[0., 0., 0.],
[0., 0., 0.]])
3. Print Range Between 1 To 15 and show 4 integers random numbers:
np.random.randint(1,15,4)
array([12, 1, 14, 9])
4. Use numpy to generate array of 25 random numbers sampled from a standard normal distribution:
np.random.rand(25)
array([0.73293502, 0.38245306, 0.52894979, 0.35896097, 0.83880269,
0.38216059, 0.11173257, 0.38598484, 0.74322228, 0.31497052,
0.32662347, 0.4081118 , 0.47730562, 0.3063162 , 0.44843887,
0.96982928, 0.50541644, 0.71667506, 0.53888407, 0.37803468,
0.74569824, 0.74611054, 0.56089105, 0.28962139, 0.5674749 ])
5. Stack horizontally the two arrays:
import numpy as np
np.arange(10).reshape(2,-1)
array([[0, 1, 2, 3, 4],
[5, 6, 7, 8, 9]])
a = np.arange(10).reshape(2,-1)
b = np.repeat(1, 10).reshape(2,-1)
np.concatenate([a, b], axis=1)
array([[0, 1, 2, 3, 4, 1, 1, 1, 1, 1],
[5, 6, 7, 8, 9, 1, 1, 1, 1, 1]])
6. Find unique value in array 1 that are not array 2:
a = np.array([1,2,3,4,5])
b = np.array([5,6,7,8,9])
np.setdiff1d(a,b)
array([1, 2, 3, 4])
Related concepts: import numpy library, create array, delete array values and insert array values, unique values, reshape array values,generate random number, randon numbers in standard normal distribution.
Dictionary:
1. Python program to find the sum of all items in a dictionary:
def returnSum(dict):
sum = 0
for i in dict.values():
sum = sum + i
return sum
# Driver Function
dict = {'a': 100, 'b':200, 'c':300}
print("Sum :", returnSum(dict))
Sum : 600
2. Delete a key in dict:
my_dict = {'case 1': 1, 'case 2': 2, 'case 3': 3}
print('Original dict is:' +str(my_dict))
my_dict.pop('case 1')
print('Updated dict is :'+ str(my_dict))
Original dict is:{'case 1': 1, 'case 2': 2, 'case 3': 3}
Updated dict is :{'case 2': 2, 'case 3': 3}
Related concepts: create dictionary, delete key-value pairs, operations in dicts
Tuple:
1. Find Maximum and Minimum K elements in Tuple:
# initializing tuple
test_tup = (5, 20, 3, 7, 6, 8)
# printing original tuple
print("The original tuple is : " + str(test_tup))
# initializing K
K = 1
# Maximum and Minimum K elements in Tuple
# Using slicing + sorted()
test_tup = list(test_tup)
temp = sorted(test_tup)
res = tuple(temp[:K] + temp[-K:])
# printing result
print("The extracted values : " + str(res))
The original tuple is : (5, 20, 3, 7, 6, 8)
The extracted values : (3, 20)
Related concepts: creating a tuple, slicing and indexing a list
Bonus:
Array is a reference type that means any operation on one variable will change that value for all properties that reference that object. To prevent that, use copy().
List can hold values of different data types whereas Array can only hold values of one data type.
Tuple is not iterable. it is widely used only where the definition of items is only once.
Map takes all objects in a list and allows you to apply a function to it.
Filter takes all objects in a list and runs that through a function to create a new list with all objects that return True in that function.
Set is initialized using {} similar to dictionary however dictionary holds key values pairs and sets do not hold duplicates.
Best Sources for practice:
Part 2 will be out next week. If you like this content, please like, share and subscribe!!
Top Viewed articles:
Comments